Frontend/React
[ 리액트(React) ] atomic - 다른 컴포넌트에서 같은 기능 꺼내 쓰기
YWTechIT
2021. 5. 10. 21:44
728x90
⚡️ 구현 팁
Atomic
구조를 구현하기 위해 Question
기능은 한 폴더에 넣어두고 두 개의 서로 다른 컴포넌트에서 Question
기능을 꺼내 쓰려고 할 때, 다음과 같이 사용 할 수 있다. 컴포넌트를 이중으로 사용할 때는 `children`을 사용해서 내부 데이터까지 넘겨주는것을 잊지말자!!!
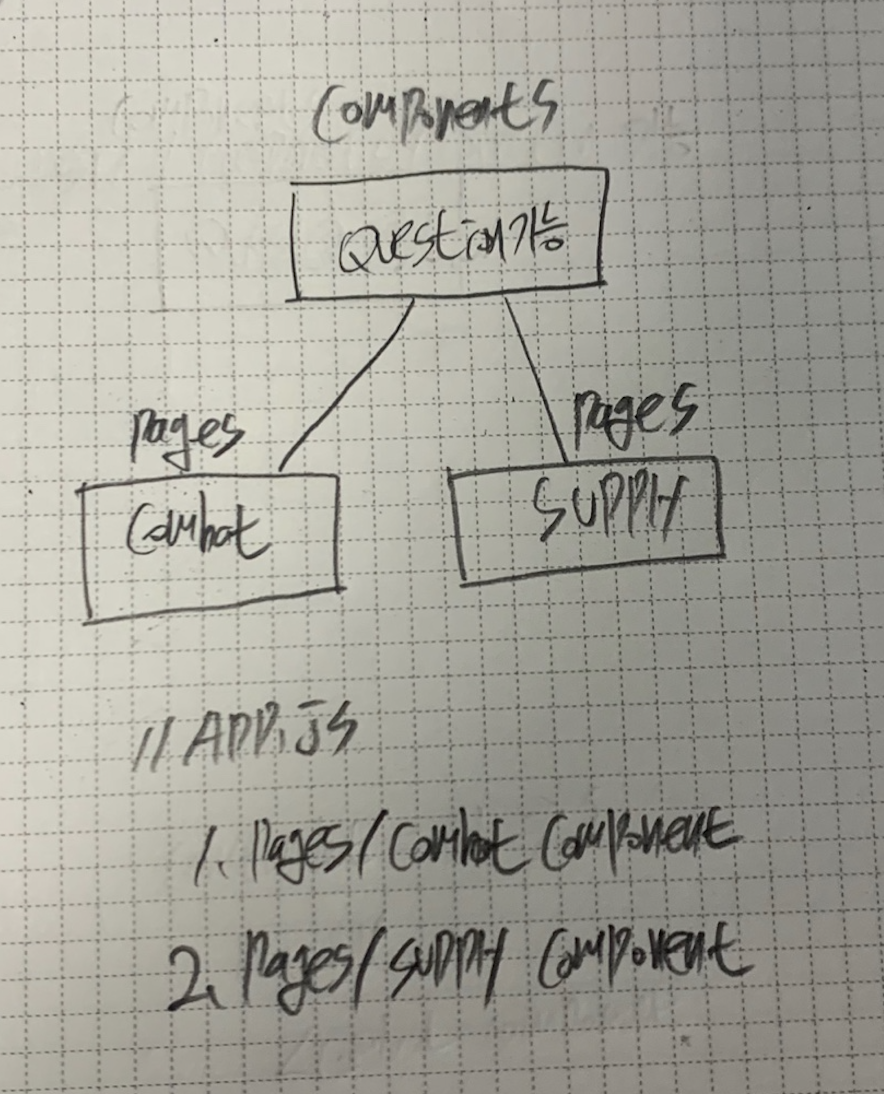
// Question 기능
// src/components/Question/index.js
import styled from "styled-components";
const QuestionWrapper = styled.div`
width: 400px;
`;
const QuestionLabel = styled.h1`
font-size: 18px;
font-weight: bold;
margin-bottom: 8px;
text-align: center;
color: grey;
`;
const QuestionTitle = styled.div`
font-size: 22px;
padding: 20px;
margin-bottom: 8px;
box-sizing: border-box;
`;
const Question = ({ QUIZ }) => (
<QuestionWrapper>
<QuestionLabel>
<span>{QUIZ[0].id}</span>/{QUIZ.length}
</QuestionLabel>
<QuestionTitle>{QUIZ[0].question}</QuestionTitle>
</QuestionWrapper>
);
export default Question;
// Question 기능을 이용하는 Page1
import react from "react";
import Question from "../../components/Quiz/Question";
const Combat = ({ QUIZ, children }) => {
return (
<>
<Question QUIZ={QUIZ}>{children}</Question>;
</>
);
};
export default Combat;
// Question 기능을 이용하는 Page2
import react from "react";
import Question from "../../components/Quiz/Question";
const Supply = ({ QUIZ, children }) => {
return (
<>
<Question QUIZ={QUIZ}>{children}</Question>;
</>
);
};
export default Supply;
// App.js
import React from "react";
import Combat from "./pages/Combat";
import Supply from "./pages/Supply";
import { COMBAT_QUIZ } from "./pages/Combat/Constant";
import { SUPPLY_QUIZ } from "./pages/Supply/Constant";
const App = () => {
return (
<>
<Combat QUIZ={COMBAT_QUIZ}></Combat>
<Supply QUIZ={SUPPLY_QUIZ}></Supply>
</>
);
};
export default App;
💡 배운 점: 같은 기능을 구현하는 코드는 중복해서 쓰지말고 한곳에서 가져다 쓰자.
반응형