๐ section09 - 1 - ๊ทธ๋ํ์ ์ธ์ ํ๋ ฌ
์ด๋ฒ ์น์
์ ๊ทธ๋ํ์ ํ์(DFS, BFS)
์ ๋ํด์ ๋ฐฐ์ด๋ค. ์ง๊ธ์ ๋ฌธ์ ๋ฅผ ํ์ง ์๊ณ ๊ทธ๋ํ์ ๋ํด ๊ฐ๋จํ๊ฒ ์์๋ณด๋ ์๊ฐ์ ๊ฐ์ ธ๋ณด์. ๊ทธ๋ํ๋ ๋ค์๊ณผ ๊ฐ์ด ์ด๋ฃจ์ด์ง๋ค.
- Vertex: ์ ์ , ๋ ธ๋
- Edge: ๋ ธ๋์ ๋ ธ๋๋ฅผ ์ฐ๊ฒฐํ๋ ๊ฐ์
- graph: 2์ฐจ์ ๋ฐฐ์ด์์๋
index
๊ฐ ์ ์ (vertex) ๋ฒํธ๋ค.
๊ทธ๋ํ์ ์ข
๋ฅ๋ 3๊ฐ์ง๊ฐ ์๋ค. ์
๋ ฅ์ด ์ฃผ์ด์ง ๋ graph
๋ณ๋ก ์ด๋ป๊ฒ ์
๋ ฅํ๋์ง ์ฝ๋๋ก ๋ํ๋๋ค. (์ฌ๊ธฐ์๋ ์ธ์ ํ๋ ฌ๋ก ํํํจ)
1. ๋ฌด๋ฐฉํฅ ๊ทธ๋ํ(unDirected Graph): ๋ฐฉํฅ์ด ์๋ ๊ทธ๋ํ ์ฆ, ์๋ฐฉํฅ์ธ ๊ทธ๋ํ๋ค. ์
๋ ฅ์ ๋ณดํต [a, b]
ํํ๋ก ์ฃผ์ด์ง๋๋ฐ ์ฌ๊ธฐ์ graph[a][b]=1, graph[b][a]=1
์ ๋ชจ๋ ์ํํ๋ค.
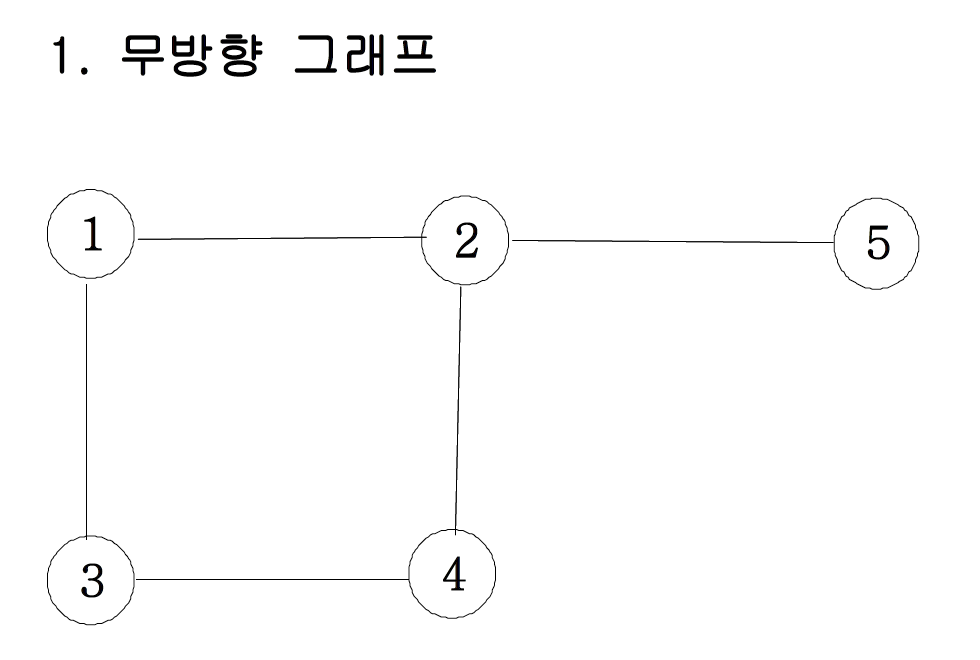
// ์ธ์ ํ๋ ฌ
let node = 5;
let edge = 5;
console.log(unDirectedGraph(node, edge));
function unDirectedGraph(node, edge){
let graph = Array.from(Array(node+1), () => Array(edge+1).fill(0));
let input = [[1, 2], [1, 3], [2, 4], [3, 4], [2, 5]];
for (let [a, b] of input){
graph[a][b] = 1;
graph[b][a] = 1;
}
return graph;
}
๐๐ฝ [
[ 0, 0, 0, 0, 0, 0 ],
[ 0, 0, 1, 1, 0, 0 ],
[ 0, 1, 0, 0, 1, 1 ],
[ 0, 1, 0, 0, 1, 0 ],
[ 0, 0, 1, 1, 0, 0 ],
[ 0, 0, 1, 0, 0, 0 ]
]
2. ๋ฐฉํฅ ๊ทธ๋ํ(directed Graph): ๋ฐฉํฅ์ด ์ ํด์ ธ ์๋ ๊ทธ๋ํ๋ค. ๋ฌด๋ฐฉํฅ ๊ทธ๋ํ์๋ ๋ฌ๋ฆฌ ์ ์ ์ด ๊ฐ๋ฆฌํค๋ ๋ฐฉํฅ๋ง graph
์ ์
๋ ฅํ๋ค.
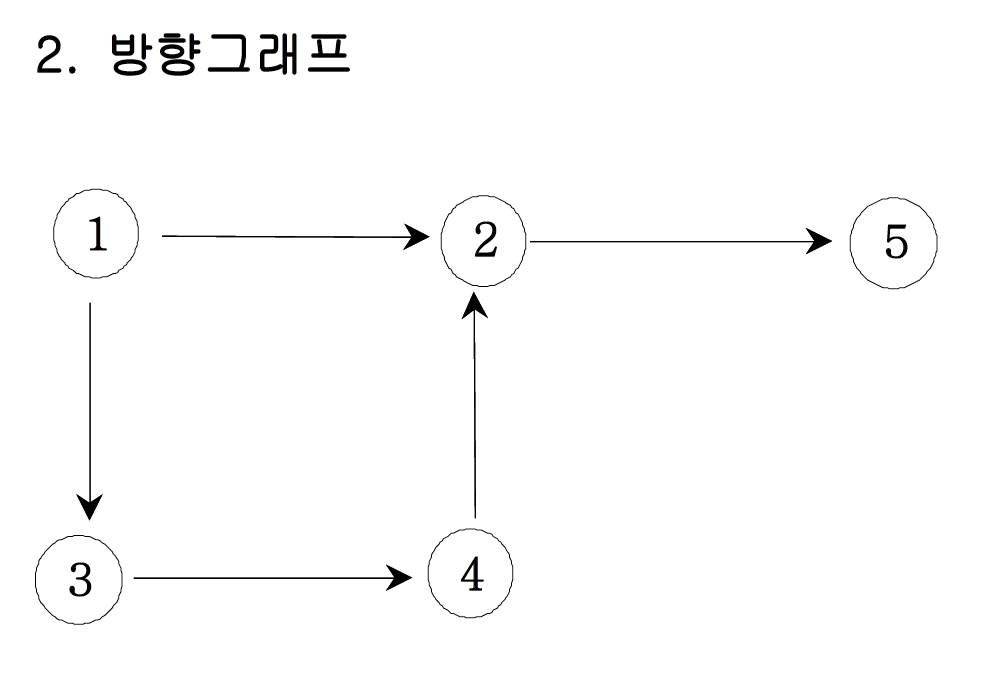
// ์ธ์ ํ๋ ฌ
console.log(directedGraph(node, edge));
function directedGraph(node, edge){
let graph = Array.from(Array(node+1), () => Array(edge+1).fill(0));
let input = [[1, 2], [1, 3], [2, 4], [3, 4], [2, 5]];
for (let [a, b] of input){
graph[a][b] = 1;
}
return graph;
}
๐๐ฝ [
[ 0, 0, 0, 0, 0, 0 ],
[ 0, 0, 1, 1, 0, 0 ],
[ 0, 0, 0, 0, 1, 1 ],
[ 0, 0, 0, 0, 1, 0 ],
[ 0, 0, 0, 0, 0, 0 ],
[ 0, 0, 0, 0, 0, 0 ]
]
3. ๊ฐ์ค์น ๋ฐฉํฅ ๊ทธ๋ํ(weighted directed Graph): ๋ฐฉํฅ ๊ทธ๋ํ์ด๋ฉด์ ๊ฐ์ค์น๋ ๊ฐ์ด ๋ํ๋ธ ๊ทธ๋ํ์ด๋ค. ํ ๋์์์ ๋ค๋ฅธ ๋์๋ก ์ด๋ํ ๋ ๋๋ ๋น์ฉ๊ณผ ๊ฐ์ ๋ฌธ์ ์์ ๋ฑ์ฅํ๋ค. ์
๋ ฅ์ a, b, c
๋ก ๋ฐ์ graph[a][b]=c
์ฒ๋ผ ๋น์ฉ๊น์ง ๊ฐ์ด graph
์ ์
๋ ฅํด์ผํ๋ค.
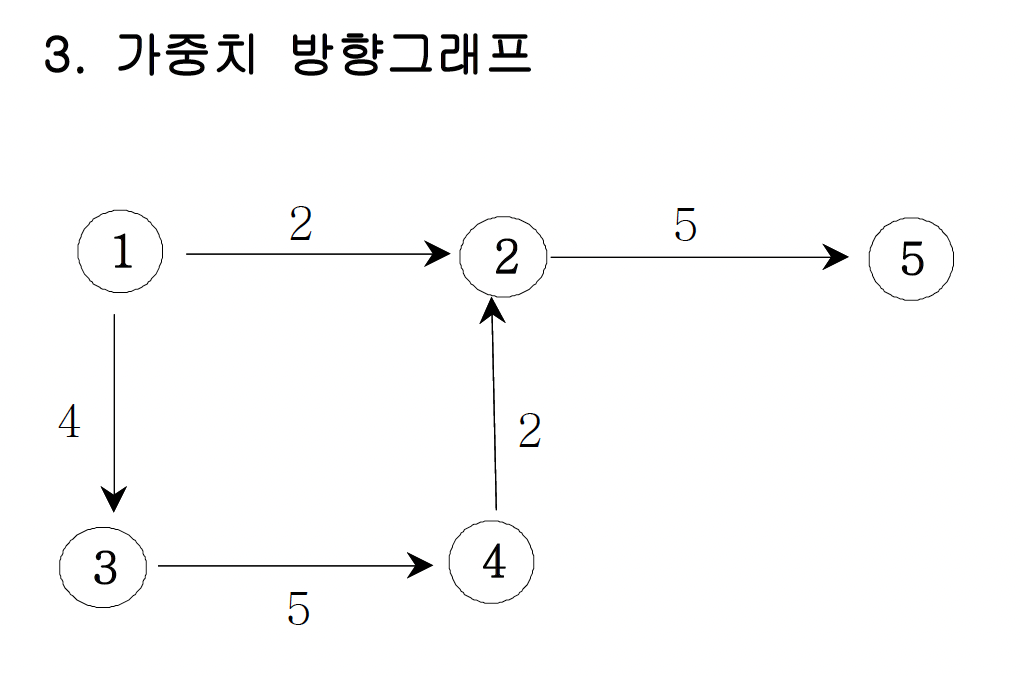
// ์ธ์ ํ๋ ฌ
console.log(weightedDirectedGraph(node, edge));
function weightedDirectedGraph(node, edge){
let graph = Array.from(Array(node+1), () => Array(edge+1).fill(0));
let input = [[1, 2, 2], [2, 5, 5], [3, 4, 5], [1, 3, 4], [4, 2, 2]];
for (let [a, b, c] of input){
graph[a][b] = c;
}
return graph;
}
๐๐ฝ [
[ 0, 0, 0, 0, 0, 0 ],
[ 0, 0, 2, 4, 0, 0 ],
[ 0, 0, 0, 0, 0, 5 ],
[ 0, 0, 0, 0, 5, 0 ],
[ 0, 0, 2, 0, 0, 0 ],
[ 0, 0, 0, 0, 0, 0 ]
]
์์์๋ ์ธ์ ํ๋ ฌ๋ก ๋ํ๋๋๋ฐ, ๊ทธ๋ํ์ ๊ฐ์ ๋ํ๋ผ ๋๋ ์ธ์ ํ๋ ฌ๊ณผ ์ธ์ ๋ฆฌ์คํธ์ ๋ฐฉ์์ด ์๋ค. ๊ฐ๊ฐ์ ํน์ง์ ๋ค์๊ณผ ๊ฐ๋ค.
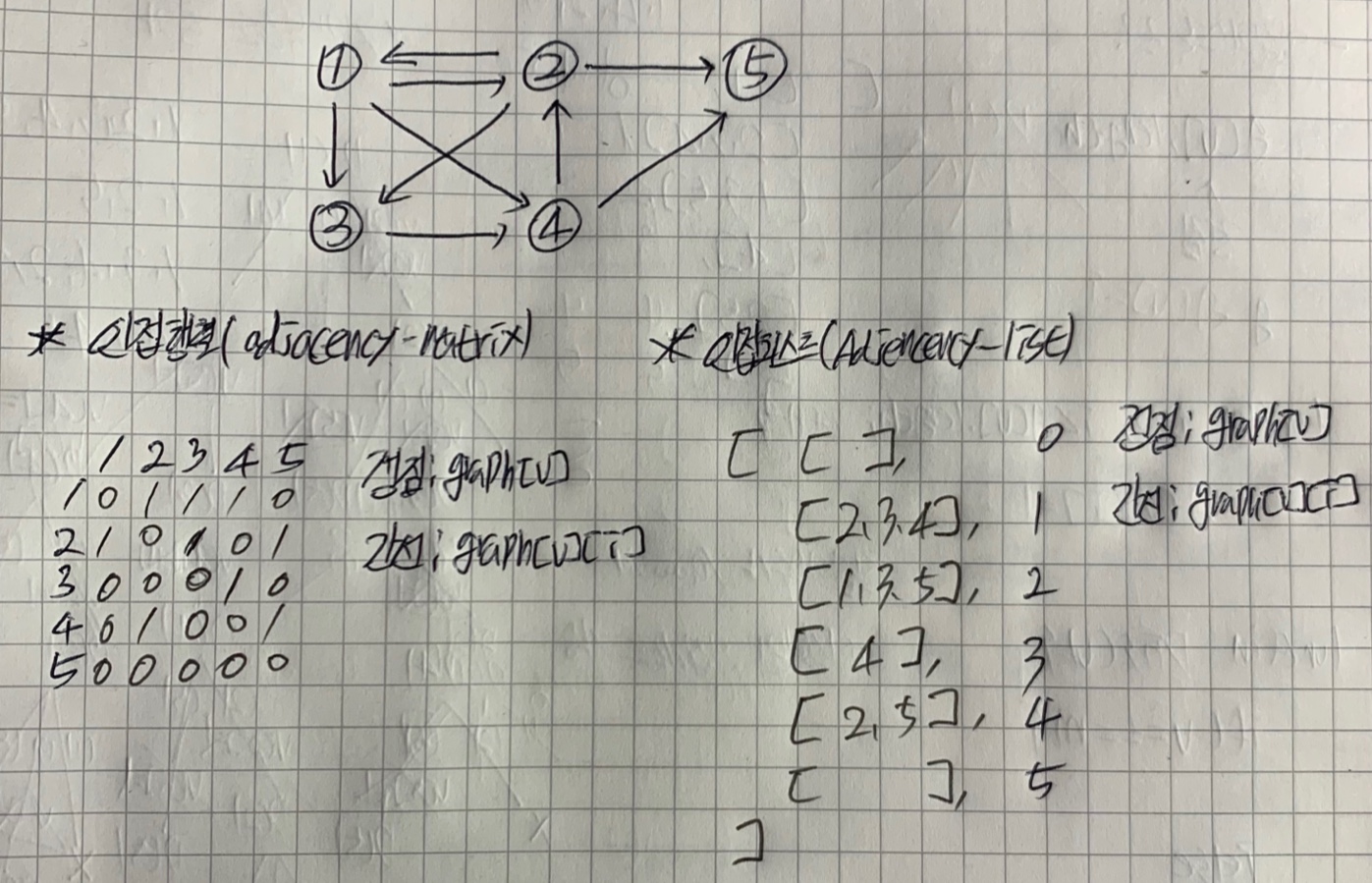
1. ์ธ์ ํ๋ ฌ(adjacency-matrix): ๋
ธ๋์ ๊ฐ์ ์ ์ ๋ณด๋ฅผ ํ๋ ฌ๋ก ํํํ๋ ๋ฐฉ๋ฒ, ๊ฐ์ ์ ๊ฐ์์ ์๊ด์์ด ๋ชจ๋ ์ ์ ์ ํํํด์ผ ํ๊ธฐ ๋๋ฌธ์ ์ ์ ์ ๊ฐ์๊ฐ ๋ง์์๋ก ๋ฉ๋ชจ๋ฆฌ ์ฌ์ฉ๋์ด ๋์ด๋๋ค. ๊ฐ์ค์น๋ฅผ ๋ฐฉํฅ๊ทธ๋ํ๋ ์ธ์ ํ๋ ฌ๋ก ๋ํ๋ธ๋ค. ๋ฌด๋ฐฉํฅ, ๋ฐฉํฅ์ฑ๊ทธ๋ํ ๋ฌธ์ ๋ฅผ ํ ๋ ์ ์ ์ ๊ฐฏ์๊ฐ 10,000
์ดํ๋ผ๋ฉด ์ธ์ ํ๋ ฌ๋ก ํ์.
// graph์ ๊ฐ์ ์
๋ ฅํ๋ ๋ฐฉ๋ฒ
let n = 5;
let m = 9;
let arr = [[1, 2], [1, 3], [1, 4], [2, 1], [2, 3], [2, 5], [3, 4], [4, 2], [4, 5]];
let graph = Array.from(Array(n+1), () => Array(n+1).fill(0));
// ๋ฌด๋ฐฉํฅ ๊ทธ๋ํ
for (let [a, b] of arr){
graph[a][b]=1;
graph[b][a]=1;
}
// ๋ฐฉํฅ ๊ทธ๋ํ
for (let [a, b] of arr){
graph[a][b]=1;
}
// ๊ฐ์ค์น ๋ฐฉํฅ ๊ทธ๋ํ
let graph = [[1, 2, 2], [2, 5, 5], [3, 4, 5], [1, 3, 4], [4, 2, 2]];
for (let [a, b, c] of arr){
graph[a][b]=c;
}
2. ์ธ์ ๋ฆฌ์คํธ(adjacency-list): ๋
ธ๋์ ๊ฐ์ ์ ์ ๋ณด๋ฅผ ๋ฆฌ์คํธ ํํ๋ก ํํํ๋ ๋ฐฉ๋ฒ, ์ฐ๊ฒฐ๋ ๊ฐ์ ๋ง ํ์ํ๊ธฐ ๋๋ฌธ์ ์ธ์ ํ๋ ฌ๋ณด๋ค ๋น๊ต์ ๊ฐ๋จํ๋ค. ์ ์ ์ ์์๋ ๊ผญ ์ค๋ฆ์ฐจ์์ด ์๋์ด๋ ์๊ด์๋ค. ์ธ์ ๋ฆฌ์คํธ๋ก ๋ฌธ์ ๋ฅผ ํ ๋๋ ์ ์ ์ ๊ฐ์๋งํผ ๋ฐ๋ณต๋ฌธ์ ๋๋ฆฌ๋๊ฒ์ด ์๋๋ผ ๊ฐ์ ์ ๊ฐฏ์ graph[v].length
๋งํผ ๋ฐ๋ณต๋ฌธ์ ๋๋ ค์ผ ์คํ ์๋๋ฅผ ๋์ผ ์ ์๋ค. ์ธ์ ๋ฆฌ์คํธ์ ์๋ ์ ์ ์ ํ์๋ก ํ ๋ฒ์ฉ ๋ค๋ ค์ผ ํ๋ ์ ์ ์ด๋ฏ๋ก (์๋ํ๋ฉด ์ด๋ฏธ ์ฐ๊ฒฐ๋์ด์๋ ์ ์ ๋ง ํ์ํ๊ธฐ ๋๋ฌธ) ์กฐ๊ฑด๋ฌธ์ graph[v][i]
๋ก ๊ฐ์ ํ์ธํ๋ ๋์ visited
์ฌ๋ถ๋ง ํ์ธํด์ฃผ๋ฉด ๋๋ค. ์ ์ ์ ๊ฐ์๊ฐ 10,000
์ด์์ผ ๋๋ ์ธ์ ๋ฆฌ์คํธ๋ฅผ ์ฌ์ฉํ์.
// graph์ ๊ฐ์ ์
๋ ฅํ๋ ๋ฐฉ๋ฒ
let n = 5;
let m = 9;
let arr = [[1, 2], [1, 3], [1, 4], [2, 1], [2, 3], [2, 5], [3, 4], [4, 2], [4, 5]];
let graph = Array.from(Array(n+1), () => Array());
// ๋ฐฉํฅ ๊ทธ๋ํ
for (let [a, b] of arr){
graph[a].push(b);
}
console.log(graph)
๐๐ฝ [ [], [ 2, 3, 4 ], [ 1, 3, 5 ], [ 4 ], [ 2, 5 ], [] ]
๋๊ธ